Enumeration (or enum) is a user defined data type in C. It is mainly used to assign names to integral constants, the names make a program easy to read and maintain.
As we have seen before in previous recipes, an enum is used to represent a collection of states. Design Patterns for Game Development. Using the singleton design pattern. Using the factory method. Using the abstract factory method. Using the observer pattern. Hangman is a popular word guessing game where the player attempts to build a missing word by guessing one letter at a time. After a certain number of incorrect guesses, the game ends and the player loses. The game also ends if the player correctly identifies all the letters of the missing word. The program consists of several. Enums or Enumerated type (enumeration) is a user-defined data type that can be assigned some limited values. These values are defined by the programmer at the time of declaring the enumerated type. Need for Enum Class over Enum Type: Below are some of the reasons as to what are the limitations of Enum Type and why we need Enum Class to cover them. The snake game is a very popular one, here is a very simple one written in C using Visual Studio. The code is only 150 line and can be modified in several ways. ///// You want to support my videos? You can browse and buy materials from my Amazon Store with the same price. This way I get a small commission: C How to Program (10th.
The keyword ‘enum’ is used to declare new enumeration types in C and C++. Following is an example of enum declaration.
Variables of type enum can also be defined. They can be defined in two ways:
// of enum in C { day = Wed; return 0; |
Output:
In the above example, we declared “day” as the variable and the value of “Wed” is allocated to day, which is 2. So as a result, 2 is printed.
Another example of enumeration is:
// Another example program to demonstrate working #include<stdio.h> enum year{Jan, Feb, Mar, Apr, May, Jun, Jul, { for (i=Jan; i<=Dec; i++) } |
Output:
In this example, the for loop will run from i = 0 to i = 11, as initially the value of i is Jan which is 0 and the value of Dec is 11.
Interesting facts about initialization of enum.
1. Two enum names can have same value. For example, in the following C program both ‘Failed’ and ‘Freezed’ have same value 0.
enum State {Working = 1, Failed = 0, Freezed = 0}; int main() printf ( '%d, %d, %d' , Working, Failed, Freezed); } |
Output:
2. If we do not explicitly assign values to enum names, the compiler by default assigns values starting from 0. For example, in the following C program, sunday gets value 0, monday gets 1, and so on.
C++ Enum Naming
enum day {sunday, monday, tuesday, wednesday, thursday, friday, saturday}; int main() enum day d = thursday; printf ( 'The day number stored in d is %d' , d); } |
Output:
3. We can assign values to some name in any order. All unassigned names get value as value of previous name plus one.
enum day {sunday = 1, monday, tuesday = 5, wednesday, thursday = 10, friday, saturday}; int main() printf ( '%d %d %d %d %d %d %d' , sunday, monday, tuesday, wednesday, thursday, friday, saturday); } |
Output:
4. The value assigned to enum names must be some integeral constant, i.e., the value must be in range from minimum possible integer value to maximum possible integer value.
5. All enum constants must be unique in their scope. For example, the following program fails in compilation.
enum result {failed, passed}; int main() { return 0; } |
Output:
Exercise:
Predict the output of following C programs
Program 1:

enum day {sunday = 1, tuesday, wednesday, thursday, friday, saturday}; int main() enum day d = thursday; printf ( 'The day number stored in d is %d' , d); } |
Program 2:
enum State {WORKING = 0, FAILED, FREEZED}; return currState; (FindState() WORKING)? printf ( 'WORKING' ): printf ( 'NOT WORKING' ); } |
Enum vs Macro
We can also use macros to define names constants. For example we can define ‘Working’ and ‘Failed’ using following macro.
#define Failed 1 |
There are multiple advantages of using enum over macro when many related named constants have integral values.
a) Enums follow scope rules.
b) Enum variables are automatically assigned values. Following is simpler
C++ Enum Count
Introduction part of this article is contributed by Piyush Vashistha. If you like GeeksforGeeks and would like to contribute, you can also write an article using contribute.geeksforgeeks.org or mail your article to [email protected] See your article appearing on the GeeksforGeeks main page and help other Geeks.
Language | ||||
Headers | ||||
Type support | ||||
Program utilities | ||||
Variadic function support | ||||
Error handling | ||||
Dynamic memory management | ||||
Date and time utilities | ||||
Strings library | ||||
Algorithms | ||||
Numerics | ||||
Input/output support | ||||
Localization support | ||||
Atomic operations(C11) | ||||
Thread support(C11) | ||||
Technical Specifications |
Basic concepts | ||||
Keywords | ||||
Preprocessor | ||||
Statements | ||||
Expressions | ||||
Initialization | ||||
Declarations | ||||
Functions | ||||
Miscellaneous | ||||
History of C | ||||
Technical Specifications |
pointer | ||||
array | ||||
bit field | ||||
atomic types(C11) | ||||
(C99) | ||||
(C11) | ||||
storage duration and linkage | ||||
external and tentative definitions | ||||
(C11) | ||||
attributes(C23) |
An enumerated type is a distinct type whose value is a value of its underlying type (see below), which includes the values of explicitly named constants (enumeration constants).
[edit]Syntax
Enumerated type is declared using the following enumeration specifier as the type-specifier in the declaration grammar:
enum attr-spec-seq(optional)identifier(optional){ enumerator-list} |
where enumerator-list is a comma-separated list (with trailing comma permitted)(since C99) of enumerator, each of which has the form:
enumeration-constantattr-spec-seq(optional) | (1) |
enumeration-constantattr-spec-seq(optional)= constant-expression | (2) |
where
identifier, enumeration-constant | - | identifiers that are introduced by this declaration |
constant-expression | - | integer constant expression whose value is representable as a value of type int |
attr-spec-seq | - | (C23)optional list of attributes,
|
As with struct or union, a declaration that introduced an enumerated type and one or more enumeration constants may also declare one or more objects of that type or type derived from it.
[edit]Explanation
Each enumeration-constant that appears in the body of an enumeration specifier becomes an integer constant with type int in the enclosing scope and can be used whenever integer constants are required (e.g. as a case label or as a non-VLA array size).
If enumeration-constant is followed by = constant-expression, its value is the value of that constant expression. If enumeration-constant is not followed by = constant-expression, its value is the value one greater than the value of the previous enumerator in the same enumeration. The value of the first enumerator (if it does not use = constant-expression) is zero.
The identifier itself, if used, becomes the name of the enumerated type in the tags name space and requires the use of the keyword enum (unless typedef'd into the ordinary name space).
Each enumerated type is compatible with one of: char, a signed integer type, or an unsigned integer type. It is implementation-defined which type is compatible with any given enumerated type, but whatever it is, it must be capable of representing all enumerator values of that enumeration.
Enumerated types are integer types, and as such can be used anywhere other integer types can, including in implicit conversions and arithmetic operators.
[edit]Notes
Unlike struct or union, there are no forward-declared enums in C:
Enumerations permit the declaration of named constants in a more convenient and structuredfashion than does #define; they are visible in the debugger, obey scope rules, and participate in the type system.
or
Moreover, as a struct or union does not establish its scope in C, an enumeration type and its enumeration constants may be introduced in the member specification of the former, and their scope is the same as of the former, afterwards.
[edit]Example
Output:
[edit]References
- C17 standard (ISO/IEC 9899:2018):
- 6.2.5/16 Types (p: 32)
C++ Enum
- 6.7.2.2 Enumeration specifiers (p: 84-85)
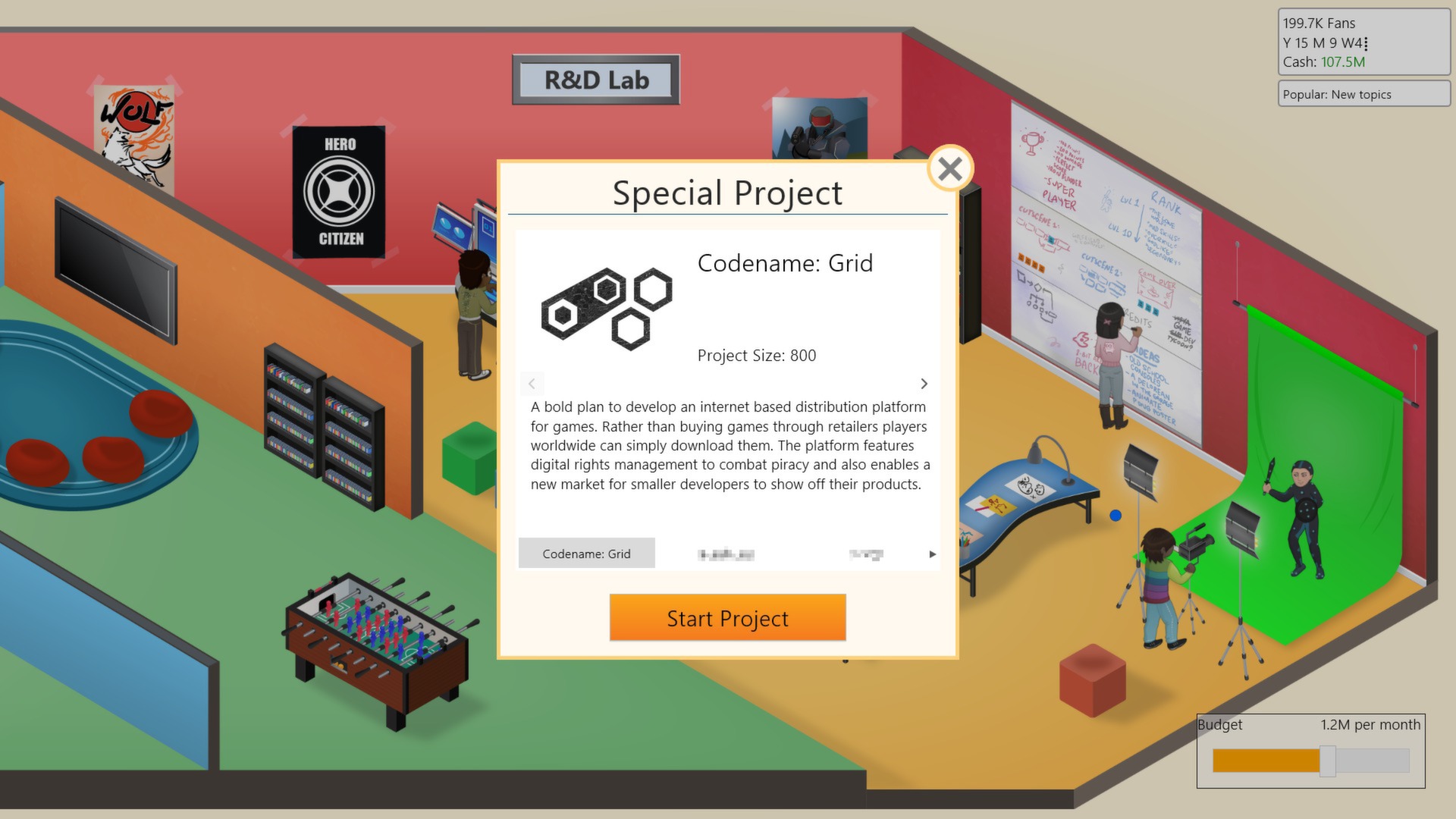
C++ Enum Class Int
- C11 standard (ISO/IEC 9899:2011):
- 6.2.5/16 Types (p: 41)
- 6.7.2.2 Enumeration specifiers (p: 117-118)
- C99 standard (ISO/IEC 9899:1999):
- 6.2.5/16 Types (p: 35)
- 6.7.2.2 Enumeration specifiers (p: 105-106)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.1.2.5 Types
C Game Dev Enumerated
- 3.5.2.2 Enumeration specifiers
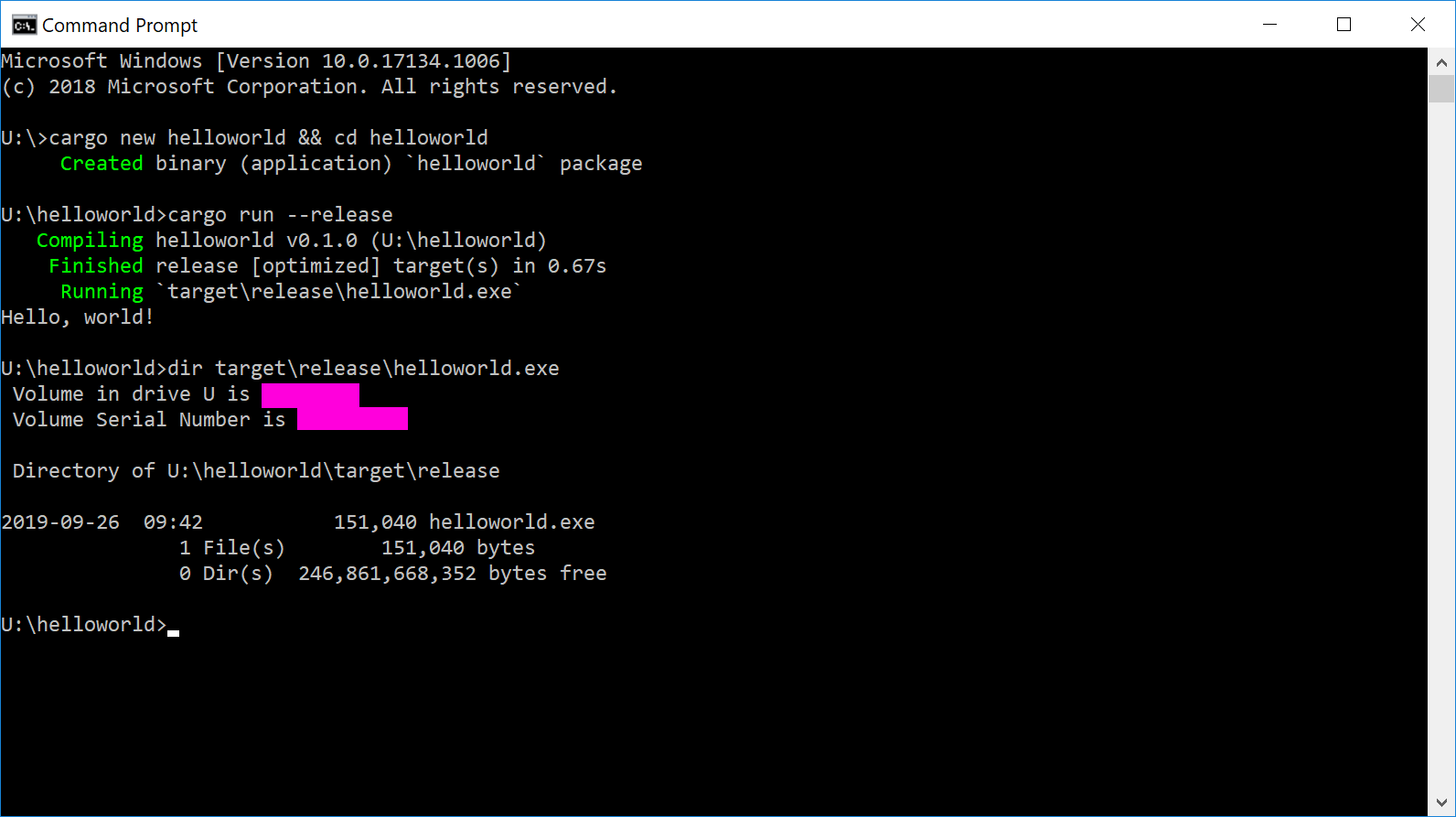
[edit]Keywords
[edit]See also
